Enforcing a consistent Coding Style across projects and programming languages
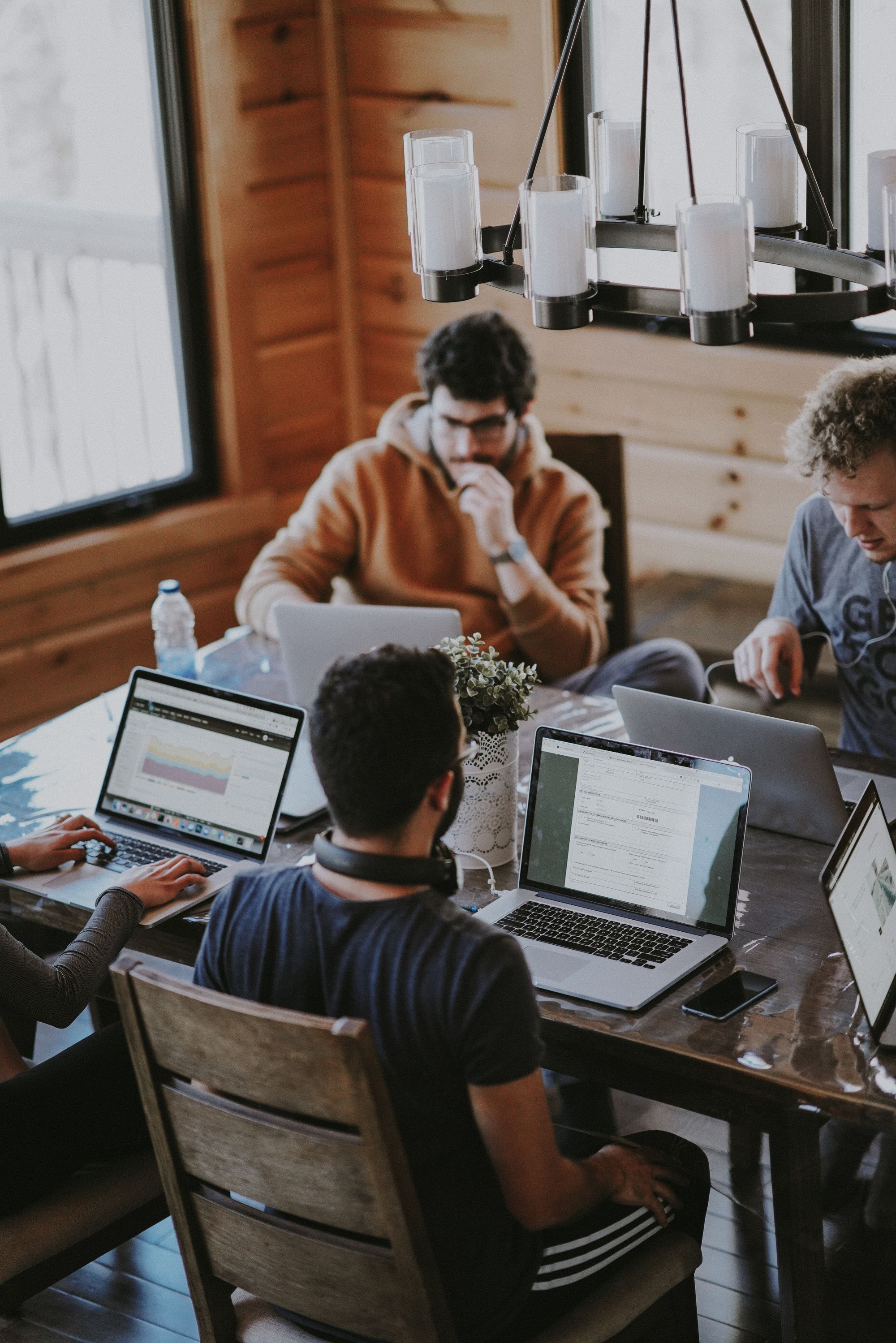
Coding with style is hard. Especially because it is a very personal and opinionated thing – as always when it comes to style. To not offend anyone in the team but still follow a consistent code format, teams should agree on a consistent set of rules and implement automatic formatters which reformat code silently, if anyone is not following these rules by accident.
Agree on this. In the past, I used to blame developers on my projects for their coding style or not following my formatting. Now I blame myself for not having implemented any rules, policies or formatters for it.
— Robin-Manuel Thiel (@robinmanuelt) March 28, 2019
We should all try to automatically force developers into succeess! https://t.co/0m7vrH5y4w
I have experienced the following challenges when it comes to these rules and formatters:
- Find formatters to re-format documents automatically
- Agree on a style per programming language
- Find a formatter that works in every environment across IDEs and platforms
- Make sure, your team is following the styling rules
The problem with formatters and styles
In 2012, Nicholas C. Zakas already wrote about the importance of Coding Styles and it is generally accepted these days to have one. But as Coding Style is such an emotional topic, discussions around the right set of rules can heat up quickly and consume a lot of time. Because of this, I always recommend opinionated code formatters, without too many configuration options. Otherwise, you will end up having discussions about how to configure the formatter.
There is no formatter to rule them all
Unfortunately, there is no formatter that works across all languages and frameworks. This is why you will most likely end up with multiples, especially if your project consists of multiple programming languages and technologies like an .NET Core Backend and Angular CLI Frontend combination for example.
Start with EditorConfig
Let’s start with very basic rules like Charset, Indentation size, Line End and so on. EditorConfig is a very good start for that. You simply add an .editorconfig
file at the root of your project and define the basic rules.
EditorConfig is widely spread and supported by the most popular editors and IDEs like Visual Studio Code, Atom, Sublime and many more. If your editor supports format on save, you will at least have unified indentations, charsets and line endings from now on across all your project files.
Below, you can find a sample .editorconfig
file for a project’s root folder.
root = true
# All files
[*]
indent_style = space
indent_size = 2
end_of_line = lf
charset = utf-8
trim_trailing_whitespace = true
insert_final_newline = true
# .NET formatting
[*.cs]
indent_size = 4
Use Prettier for HTML, CSS, JS, TS
One of those opinionated formatters I mentioned earlier is Prettier. Like EditorConfig, it supports a wide variety of editors and languages and offers a community defined set of coding styles (over 30.000 stars on GitHub).
Something about prettier I had to get used to, is the placement of the closing >
in htmlelements. But once I read the explanation, I was understood why it is the best solution.
Prettier can be customized with a small set of options which get defined in a .prettierrc.yaml
file. Nothing too specific, just enough to define the basic look and feel of your code. Things like quote-style and max-width are two examples. Especially max-width is important, as Prettier does smart line breaks when your code exceeds the desired width.
Below, you can find a sample .prettierrc.yaml
file for a project’s root folder.
printWidth: 100
singleQuote: true
htmlWhitespaceSensitivity: "ignore"
Prettier supports many languages out of the box and others via community plugins. I strongly recommend using Prettier for every language it supports.
Use official coding convention settings for .NET
As .NET is not supported by prettier yet, I recommend the official .NET coding convention settings, which get picked up by Visual Studio (the most common .NET IDE) automatically.
The good news: .NET coding conventions also can be defined in .editorconfig
file! So we can declare the set of .NET rules there as well. Below, you can find a sample .editorconfig
file with .NET formatting rules.
[*]
# ...
# .NET formatting
[*.cs]
indent_size = 4
csharp_new_line_before_open_brace = all
csharp_indent_case_contents = true
csharp_indent_switch_labels = true
csharp_space_after_cast = false
I haven’t found anything more opinionated than that, but you can follow the official recommendations, and implement the corresponding rules if you want to avoid discussions with your team.
Make sure, your team uses the formatters
One of the best ways to onboard new developers is to force them into success. In case of coding style, this means that the editor automatically formats the written code into the desired state when a document is saved. For both, EditorConfig and Prettier there are plugins for the most common editors out there. .NET is a little bit different but the support is already there for Visual Studio and is coming for Visual Studio Code.
Speaking of Visual Studio Code: As this is the main editor in most of my teams, I also add a .vscode
folder with a settings.json
and extensions.json
file to the repository. They can define the preferred settings (like format on save) and wanted or unwanted extensions for a project. Here you can add the extensions you prefer for your code formatting and also ban those you don’t.
Checking Pull Requests for Coding Style violations in your Build Pipelines
To enforce and keep a consistent Coding Style, I add corresponding checks to those Build Pipelines in my CI/CD system, that check Pull Requests. This not only ensures that the code base also follows my style, it’s also another way to force developers into success, as they get an immediate feedback from the build system, if they didn’t follow any of the defined rules.
Install Code Formatters
For all the formatters I have introduced in this post, there are command line tools available, to check the code for style violations like eclint, dotnet-format and prettier. Within my Pull Request check scripts, I also include the following commands:
npm install -g eclint
dotnet tool install -g dotnet-format
npm install -g prettier
# Check for Coding Style violations
eclint check $(git ls-files)
dotnet-format --dry-run --check
prettier --check "**/*.{js,ts,html,css,scss,md,json}"
This script lets the build fail, if any Coding Style rules have been violated and the developer can fix them, before the code review begins.
If you are using Azure DevOps as your CI/CD system like I do, check out my other article, which describes how to setup a pipeline in Azure DevOps that checks for Coding Style violations.
So let us use these weapons to fight together for clean code and consistent Coding Styles in our projects! For our queen, the OCD!
☝️ Advertisement Block: I will buy myself a pizza every time I make enough money with these ads to do so. So please feed a hungry developer and consider disabling your Ad Blocker.